Introduction to Svelte's Reactivity System
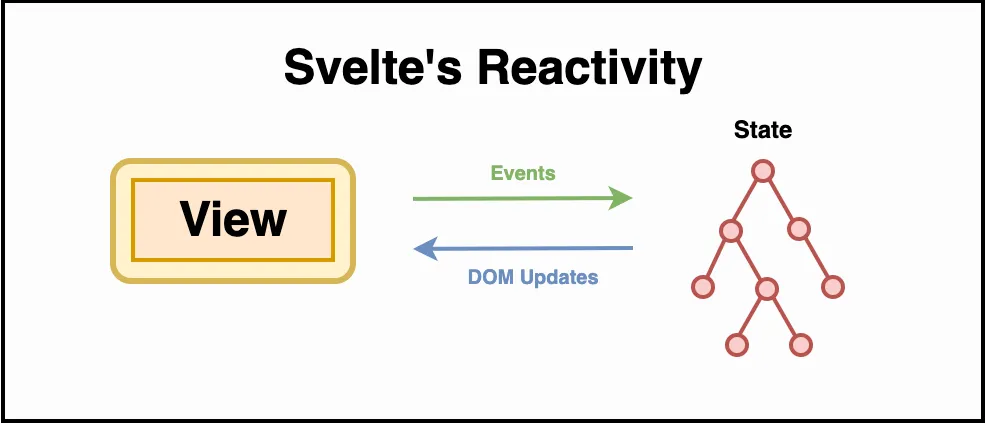
Concept of Reactivity
Reactivity is an essential concept that has become common with modern frontend frameworks. This concept allows the user interface (UI) to update automatically when the application has any state changes. Svelte provides different methods to implement reactivity.
Types of Reactivity in Svelte:
Variables are the most basic form of reactivity in Svelte. When the value is changed, the UI updates accordingly.
Let’s have an array of frameworks and add a new framework to the array, and in a list, we will display all the frameworks.
<script>
let frameworks = ["React", "Vue", "Angular"];
function addFramework(name) {
frameworks.push(name); // reactivity didn't work, why?
}
</script>
<button on:click={() => addFramework("svelte")}>add framework</button>
<ul>
{#each frameworks as f}
<li>{f}</li>
{/each}
</ul>
Firstly, svelte
is added to the array but the UI didn’t update. Why?
Because the push
method didn’t create a new reference, it just modifies the original array. Svelte needs a new reference to detect the change and trigger reactivity.
Here is the solution for reactivity to work:
We will use the spread
operator to create a new array instead of the push
method. And then reactivity will be triggered.
<script>
let frameworks = ["React", "Vue", "Angular"];
function addFramework(name) {
frameworks = [...frameworks, name]; // reactivity works
}
</script>
<button on:click={() => addFramework("svelte")}>add framework</button>
<ul>
{#each frameworks as f}
<li>{f}</li>
{/each}
</ul>
Unlike other frameworks, Svelte automatically updates the UI when a variables changes. This makes the application faster and more efficient.
For instance, in React, when you change the value of a variable using the setState
function, the component needs to be rendered again.
Reactive statements are the lines of code that are executed when the value of a variable changes. This is useful when you want to perform some action when a variable changes.
<script>
let isDarkMode = false;
$: {
if (isDarkMode) {
document.body.classList.add('dark');
} else {
document.body.classList.remove('dark');
}
}
</script>
<label>
<input type="checkbox" bind:checked={isDarkMode}>
Toggle Dark Mode
</label>
<style>
.dark {
background-color: #333;
color: #fff;
}</style>
For example, in the example above, a reactive statement is used to add or remove the class of the document.body element when the value of the isDarkMode variable is changed.
The $:
sign marks the beginning of a reactive statement
. This statement is executed when the value of the isDarkMode
variable changes and adds or removes the class of the document.body
element.
Reactive declarations are used to create reactive values that depend on other reactive values. For example, you can create a reactive value that is calculated based on the value of another reactive value.
Let’s demonstrate this with an example:
<script>
let initialRelease = new Date("26/November/2016");
let currentDate = new Date();
$: seconds = Math.floor((currentDate - initialRelease) / (1000));
</script>
<button on:click={() => currentDate = new Date()}>Calculate</button>
<p>{seconds} seconds have passed since Svelte first released.</p>