WordPress Site Speed Optimization: Headless WordPress and SvelteKit Integration
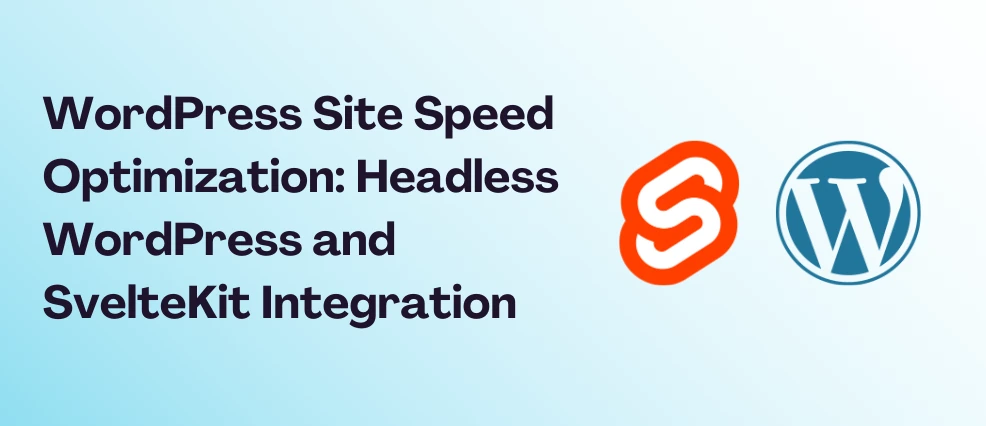
What is WordPress and Why Use It?
WordPress is the most widely used open-source content management system (CMS) globally for creating websites and blogs. Over 40% of websites on the internet utilize the WordPress platform.
However, the increasing performance and flexibility needs of modern web applications are driving developers to seek new solutions. In this context, the integration of SvelteKit and headless WordPress stands out.
In this article, we will explore how these two technologies can be implemented together and the benefits they bring to web development.
Advantages of Headless WordPress
- Flexibility: By combining WordPress with SvelteKit, you can create customized web applications.
- Performance: Separating the backend and frontend allows for faster page loads, content delivery via CDN, and the use of caching, resulting in performance improvements.
- Security: With a Reverse Proxy for DDoS protection, CORS for secure API requests, and JWT (JSON Web Tokens) for authentication, you can implement robust security measures.
- Scalability: Easily respond to traffic increases and better manage resource optimization.
Powerful Features of SvelteKit
- Rapid Development: Svelte’s simple and effective code structure provides developers with a faster development experience.
- Performance: As a compile-time framework, Svelte ensures high performance with minimal JavaScript code.
- SEO Friendly: SvelteKit’s server-side rendering (SSR) and static site generation (SSG) capabilities enable search engine bots to crawl content more effectively. Additionally, managing dynamic meta tags and integrating structured data becomes easier.
- Flexible Routing System: With file-based routing, you can quickly create URL structures and easily manage complex routes.
- Adapter-Based Deployment: SvelteKit offers various adapters for different hosting platforms (Vercel, Netlify, Node.js, static hosting). This allows you to deploy and scale your project across different environments effortlessly.
Integrating SvelteKit and Headless WordPress
In this section, we will go through a step-by-step process of integrating SvelteKit with headless WordPress. We will start by converting WordPress to headless mode and then use this data through SvelteKit.
1. Switching WordPress to Headless Mode
First, you need to switch WordPress to headless mode. To do this:
- Enable the REST API: The WordPress REST API is enabled by default.
- Configure CORS Settings: Adjust Cross-Origin Resource Sharing (CORS) settings to allow SvelteKit to communicate with the WordPress API.
- JWT Authentication: Add JWT authentication for user authentication and secure API requests.
2. Creating a SvelteKit Project
To create a SvelteKit project, follow these steps in your terminal:
npm create svelte@latest wp-sveltekit-app
cd wp-sveltekit-app
npm install
3. Communicating with the WordPress API
Create a $lib/api.js
file: This file will fetch posts from WordPress and make them ready for use in your SvelteKit application.
const WP_API_URL = 'https://your-wordpress-site.com/wp-json/wp/v2';
export async function fetchPosts(page = 1, perPage = 10) {
const response = await fetch(`${WP_API_URL}/posts?_embed&page=${page}&per_page=${perPage}`);
const posts = await response.json();
return posts;
}
export async function fetchPost(postId) {
const response = await fetch(`${WP_API_URL}/posts?slug=${postId}&_embed`);
const posts = await response.json();
return posts[0];
}
4. Blog Posts Page
On our blog page, we will list the posts fetched from the WordPress REST API. First, create a blog
folder under the routes
directory.
Then, create the src/routes/blog/+page.js
file as follows:
import { fetchPosts } from "$lib/api";
export async function load() {
try {
// We don't use await here because Svelte will manage the request on the UI side with the {#await} block
const posts = fetchPosts();
return {
posts,
};
} catch (error) {
console.error("Error loading posts:", error);
return {
posts: [],
error: "Unable to load posts.",
};
}
}
Edit the src/routes/blog/+page.svelte
file as follows:
<script>
export let data;
const posts = data && data.posts;
</script>
<main>
<h1>Latest Posts</h1>
{#await posts}
<p>Loading...</p>
{:then posts}
{#if posts.length === 0}
<p>No posts to display.</p>
{:else}
{#each posts as post}
<article>
<h2><a href="/blog/{post.slug}">{post.title.rendered}</a></h2>
<div>{@html post.excerpt.rendered}</div>
</article>
{/each}
{/if}
{:catch error}
<p>Error: {error.message}</p>
{/await}
</main>
5. Post Detail Page
Here, we will create a page that displays the detailed content of each blog post. First, create a [postId]
folder under the blog
directory for dynamic routing.
Create the src/routes/blog/[postId]/+page.js
file as follows:
import { fetchPost } from '$lib/api';
export async function load({ params }) {
const post = await fetchPost(params.postId);
return {
post
};
}
Edit the src/routes/blog/[postId]/+page.svelte
file as follows:
<script>
import { page } from "$app/stores";
import { fetchPost } from "$lib/api";
let post;
$: fetchPost($page.params.postId).then((data) => post = data);
</script>
{#if post}
<article>
<h1>{post.title.rendered}</h1>
<div>{@html post.content.rendered}</div>
</article>
{:else}
<p>Loading...</p>
{/if}
6. Dynamic Meta Tags for SEO
On each post page, we will add the post title and description to the meta tags to improve SEO performance. To do this, define meta information in the +page.js
file.
Edit the src/routes/blog/[postId]/+page.js
file:
import { fetchPost } from '$lib/api';
export async function load({ params }) {
const post = await fetchPost(params.postId);
return {
post,
// Sending meta information to the page
meta: {
title: post.title.rendered,
description: post.excerpt.rendered.replace(/<[^>]*>/g, '')
}
};
}
Add meta tags to the src/routes/blog/[postId]/+page.svelte
file using the svelte:head
tag:
<script>
import { page } from "$app/stores";
import { fetchPost } from "$lib/api";
let post;
let title = ""; // Title
let description = ""; // Description
$: fetchPost($page.params.postId).then((data) => {
post = data;
// Extracting title and description from the fetched data
title = post.title.rendered;
description = post.excerpt.rendered.replace(/<[^>]*>/g, "");
});
</script>
<!-- Adding title and description information using svelte:head tags -->
<svelte:head>
<title>{title}</title>
<meta name="description" content={description} />
</svelte:head>
This way, by adding dynamic titles and descriptions for each post, you enhance your SEO performance.
Conclusion
The integration of SvelteKit and headless WordPress offers a powerful and flexible solution in the modern web development landscape. This combination merges the rich content management features of WordPress with the performance and scalability of SvelteKit, enabling you to develop fast, secure, and SEO-friendly web applications.
The advantages you gain from this integration include:
- Faster page loads
- Improved user experience
- Enhanced SEO performance
- More secure applications
This article covers the first steps in developing modern web applications with SvelteKit and headless WordPress. You can extend this integration with more customization, user authentication, or custom content types.
By solving speed issues that cannot be resolved with WordPress plugins using SvelteKit, you can benefit from our Svelte and SvelteKit Consulting service for high-performance, fast, secure, and SEO-friendly websites.
You can contact us for free consultation and support.
Book Free Consultation